To design a simple digital input-output system using a push button and a buzzer on a Raspberry Pi Pico. The goal is to toggle the buzzer ON and OFF each time the button is pressed.
Buy Raspberry Pi Pico Basic Kit
Items Required:
Raspberry Pi Pico 1
Push Button 1
Buzzer (Piezo or active) 1
Resistor (10kO) 1
Breadboard 1
Jumper Wires As needed
USB Micro B cable 1
Computer with Thonny IDE 1
Connections:
- Button
- One end to GPIO 4 (Pin 6 on Pico)
- Other end to GND
- Pull-up resistor (internal) enabled in code
- Buzzer
- Positive terminal to GPIO 16 (Pin 21 on Pico)
- Negative terminal to GND
Connections:
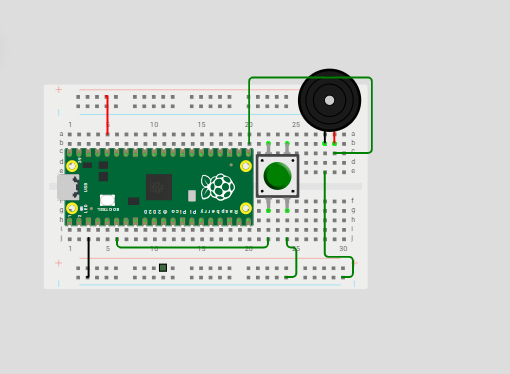
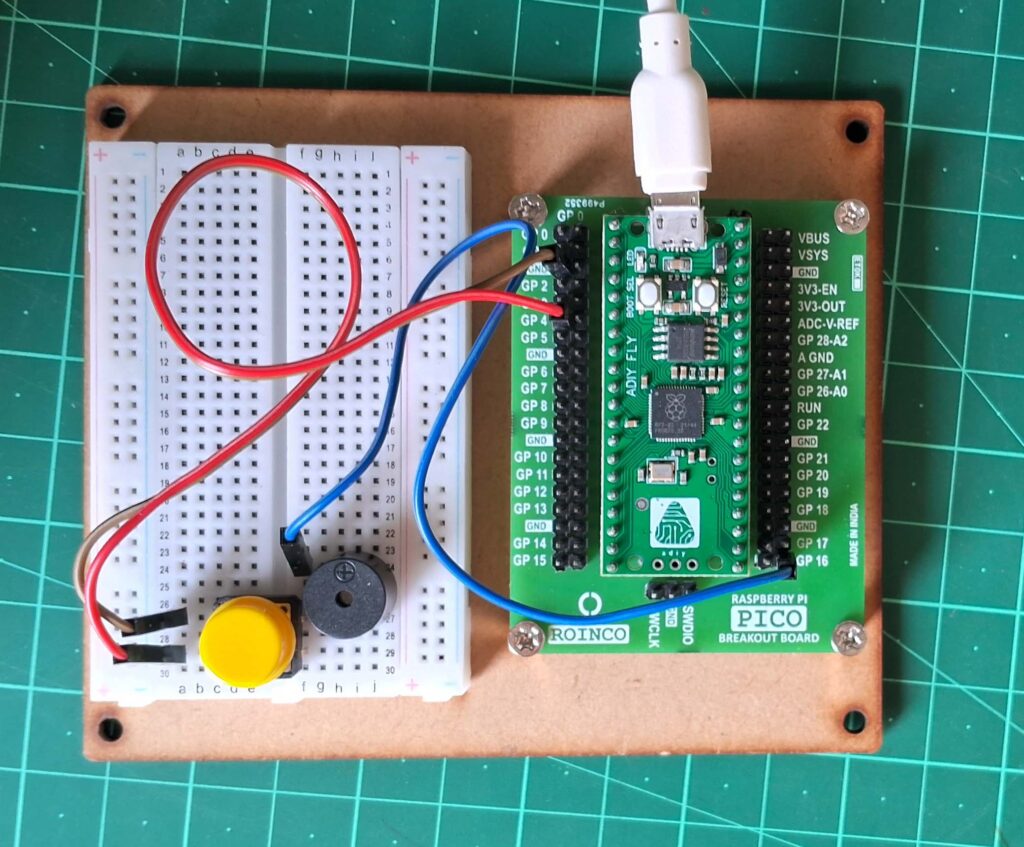
Code:
from machine import Pin
import time
button = Pin(4, Pin.IN, Pin.PULL_UP)
Buzzer = Pin(16, Pin.OUT)
Buzzer_state = False
last_button_state = 1
while True:
current_state = button.value()
if last_button_state == 1 and current_state == 0:
Buzzer_state = not Buzzer_state
Buzzer.value(Buzzer_state)
print("Buzzer ON" if Buzzer_state else "Buzzer OFF")
time.sleep(0.2) # Debounce delay
last_button_state = current_state
time.sleep(0.01) # Polling delay
Result:
- Initially, the buzzer remains OFF.
- Each time the button is pressed and released (detected as a falling edge), the buzzer toggles its state.
- If it was OFF, it turns ON with a sound. If it was ON, it turns OFF.
- The current state (“Buzzer ON” or “Buzzer OFF”) is printed in the console for verification.